Table of Contents
In this tutorial, you’ll learn about Arrays in Java, Declaring and Initializing Arrays, Accessing Array Elements, Common Operations along with example.
- An array in Java is a container object that holds a fixed number of elements of the same type.
- Each element in an array is identified by its index, starting from 0.
- Arrays provide a convenient way to work with collections of data, such as a list of numbers or a set of names.
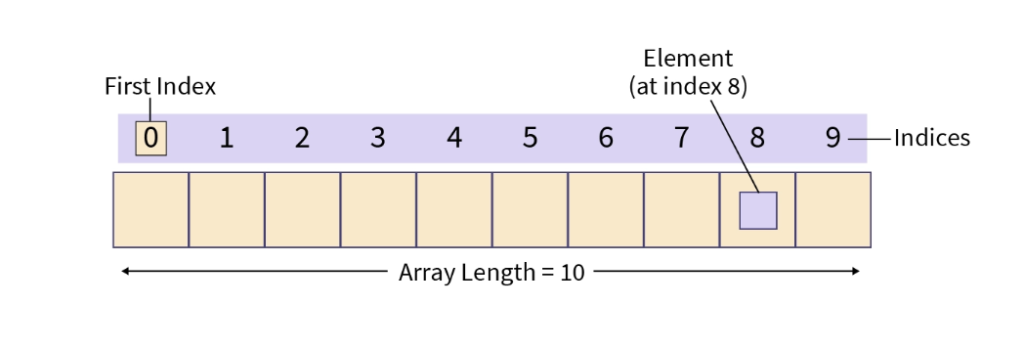
Declaring and Initializing Arrays
1. Declaration:
To declare an array, you specify the type of elements it will hold, followed by square brackets []
:
// Declare an array of integers
int[] numbers;
2. Initialization:
You can initialize an array using the new
keyword:
// Initialize an array of integers with a size of 5
numbers = new int[5];
Alternatively, you can combine declaration and initialization in a single line:
// Declare and initialize an array of integers with a size of 5
int[] numbers = new int[5];
Accessing Array Elements
Array elements are accessed using their index, with the first element having an index of 0.
int firstElement = myArray[0];<br>System.out.println("First Element: " + firstElement);
// Outputs: First Element: 1
You can also modify elements by assigning new values using their index.
myArray[0] = 10;
System.out.println("Modified First Element: " + myArray[0]); // Outputs: Modified First Element: 10
Array Initialization with Values:
You can also initialize an array with specific values:
// Initializing an array with values
int[] numbers = {1, 2, 3, 4, 5};
Common Operations
1. Iterating Through an Array:
You can iterate over array elements using a for loop or an enhanced for loop.
- Using a For Loop
for (int i = 0; i < myArray.length; i++) {
System.out.println("Element at index " + i + ": " + myArray[i]);
}
- Using an Enhanced For Loop
for (int element : myArray) {
System.out.println("Element: " + element);
}
2. Finding the Length of an Array:
The length
property provides the number of elements in an array:
int arrayLength = numbers.length;
Example: Calculating the Sum of Array Elements
Let’s create a simple program that calculates the sum of elements in an array:
public class ArraySum {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
// Calculate the sum
int sum = 0;
for (int i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
// Print the result
System.out.println("Sum of elements: " + sum); // Output: Sum of elements: 15
}
}
Example
Here is an example of a Java program that takes user input to fill an array and then displays the elements entered by the user. This example uses the Scanner class to read input from the console.
import java.util.Scanner;
public class ArrayUserInput {
public static void main(String[] args) {
// Create a Scanner object to read input from the console
Scanner scanner = new Scanner(System.in);
// Ask the user for the number of elements in the array
System.out.print("Enter the number of elements in the array: ");
int n = scanner.nextInt();
// Declare and create the array with the specified size
int[] userArray = new int[n];
// Prompt the user to enter elements
System.out.println("Enter " + n + " elements:");
// Read elements from the user
for (int i = 0; i < n; i++) {
System.out.print("Element " + (i + 1) + ": ");
userArray[i] = scanner.nextInt();
}
// Display the elements entered by the user
System.out.println("You entered the following elements:");
for (int i = 0; i < userArray.length; i++) {
System.out.println("Element at index " + i + ": " + userArray[i]);
}
// Close the scanner
scanner.close();
}
}
Output
Enter the number of elements in the array: 5
Enter 5 elements:
Element 1: 2
Element 2: 3
Element 3: 5
Element 4: 7
Element 5: 9
You entered the following elements:
Element at index 0: 2
Element at index 1: 3
Element at index 2: 5
Element at index 3: 7
Element at index 4: 9