Table of Contents
In this tutorial, you will learn about the STL stack with the help of examples.
The STL stack
provides the functionality of a stack data structure in C++ which follows the LIFO (Last In First Out) principle. That’s means, the element added last will be removed first.
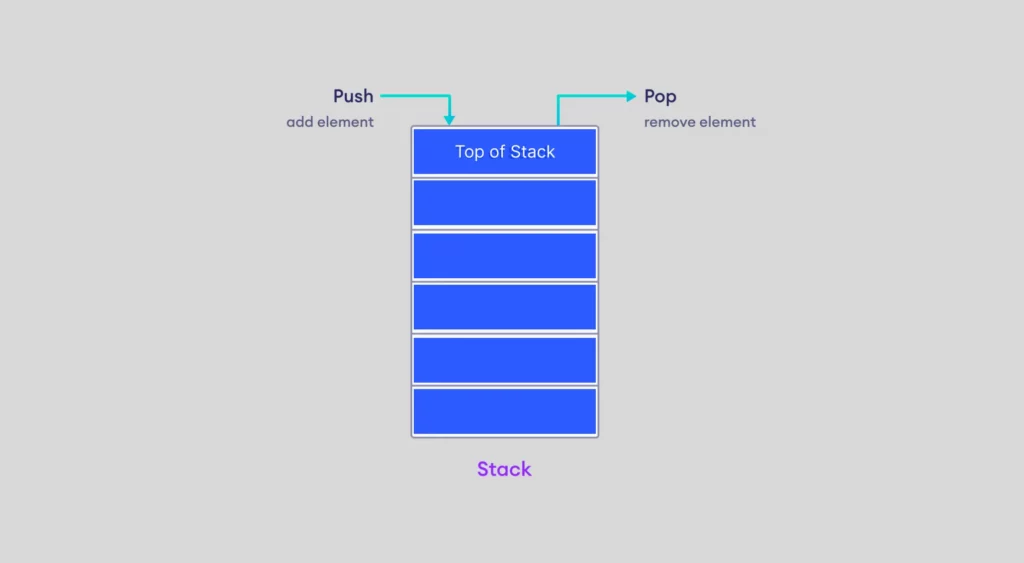
How to Create a Stack
It simple, to create a stack in C++, we first need to include the stack
header file.
#include<stack>
Once we included the file, we can create a stack
using the following syntax
stack<type> st;
Above, type indicates the datatype we want to store in the stack. For instance,
// create a stack of integers
stack<int> integer_stack;
// create a stack of strings
stack<string> string_stack;
Stack Methods
In C++, the stack
class provides various methods to perform different operations on a stack. The following methods are as follows
Operation | Description |
---|---|
push() | adds an element into the stack |
pop() | removes an element from the stack |
top() | returns the element at the top of the stack |
size() | returns the number of elements in the stack |
empty() | returns true if the stack is empty |
Let’s Explore each method one by one.
Add Element Into the Stack
We use the push()
method to add an element into a stack. For example,
#include <iostream>
#include <stack>
using namespace std;
int main() {
// create a stack of strings
stack<string> fruits;
// push elements into the stack
fruits.push("Apple");
fruits.push("Orange");
cout << "Stack: ";
// print elements of stack
while(!fruits.empty()) {
cout << fruits.top() << ", ";
fruits.pop();
}
return 0;
}
Output
Stack: Orange, Apple,
In the above code, we have used insert()
method to add the element in fruits
stack and used top()
method to view the upper most element of the stack.
Remove Elements From the Stack
We can remove an element from the stack using the pop()
method. For example,
#include <iostream>
#include <stack>
using namespace std;
int main() {
// create a stack of strings
stack<string> fruits;
// push elements into the stack
fruits.push("Apple");
fruits.push("Orange");
fruits.push("Banana");
cout << "Initial Stack: ";
// print elements of stack
display_stack(fruits);
// removes "Blue" as it was inserted last
fruits.pop();
cout << "Final Stack: ";
// print elements of stack
display_stack(fruits);
return 0;
}
// utility function to display stack elements
void display_stack(stack<string> st) {
while(!st.empty()) {
cout << st.top() << ", ";
st.pop();
}
cout << endl;
}
Output
Initial Stack: Orange, Apple, Banana, Final Stack: Orange, Apple,
In the above code, we have used the pop()
method to remove an element from the stack.
Initially, the contents of the stack are {"Orange", "Apple" , Banana"}
.
Then we have used the pop()
method to remove the element.
Hence, the final stack becomes {"Orange", "Apple"}
.
Get the Size of the Stack
We use the size()
method to get the number of elements in the stack
. For example,
#include <iostream>
#include <stack>
using namespace std;
int main() {
// create a stack of int
stack<int> prime_nums;
// push elements into the stack
prime_nums.push(2);
prime_nums.push(3);
prime_nums.push(5);
prime_nums.push(7)
// get the size of the stack
int size = prime_nums.size();
cout << "Size of the stack: " << size;
return 0;
}
Output
Size of the stack: 4
In the above example, we have created a stack of integers called prime_nums
and added four elements to it.
Then we have used the size()
method to find the number of elements in the stack , prime_nums.size()
return 4.
Check if the Stack Is Empty
We use the empty()
method to check if the stack is empty. This method returns:
- 1 (true) – if the stack is empty
- 0 (false) – if the stack is not empty
#include <iostream>
#include <stack>
using namespace std;
int main() {
// create a stack of double
stack<double> nums;
cout << "Is the stack empty? ";
// check if the stack is empty
if (nums.empty()) {
cout << "Yes" << endl;
}
else {
cout << "No" << endl;
}
cout << "Pushing elements..." << endl;
// push element into the stack
nums.push(2.3);
nums.push(9.7);
cout << "Is the stack empty? ";
// check if the stack is empty
if (nums.empty()) {
cout << "Yes";
}
else {
cout << "No";
}
return 0;
}
Output
Is the stack empty? Yes Pushing elements... Is the stack empty? No
In the above code, we have used the empty()
method to determine if the stack
is empty. Initially, the stack has no elements in it. So nums.empty()
returns true
. Then we have added some elements to the stack. Again, we use nums.empty()
to determine if the stack is empty. This time, it returns false
.