Table of Contents
In this tutorial, we will learn about variables, literals, and constants in C++ with the penalty of examples.
To store your data you need something and a proper naming convention is required to differentiate between them, that’s why C++ have Variables, Literals and Constants.
What is Variables?
Variables is a storage area to hold data in computer memory. It is similar like container that we used in kitchen to store different spices and pulses, etc.
To indicate the storage area, each variable should be given a unique name (identifier), like we use to give to different container in kitchen i.e. Haldi (Turmeric) container, Rice container and more.
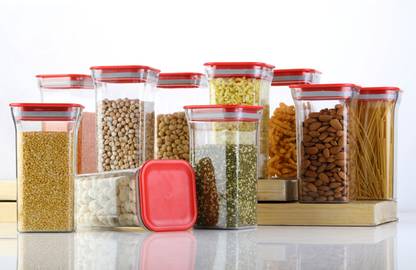
For Example,
int score=4;
Here, score is a variable of the int
data type, and we have holds an integer value 4 to it. int is refer to integer data type means, variable can hold integer values.
The value of a variable can be changed, that’s why they named as variable.
You can give variable anyname as of your choice, but there are certain rules that you need to follow.
Rules for naming a variable
- A variable name can only have alphabets, numbers, and the underscore
_
. - A variable name cannot begin with a number.
- It is best practice to begin variable names with a lowercase character. For example, name is best to be use Name.
- A variable name cannot be a keyword. For example,
int
is a keyword that can’t be used and it denote integer . - A variable name can start with an underscore. However, it’s not considered a good practice.
//A variable name should not contain space.
int data Len; (incorrect)
int dataLen; (correct)
//A variable name cannot start with a digit but you can use it between or end of the identifier.
int 2length; (incorrect)
int le2ngth; (correct)
//You cannot use a special character in identifiers except the underscore.
int len@gth; (incorrect)
int le#ngth ; (incorrect)
int len_gth; (correct)
int _length; (correct)
//A variable name cannot be a keyword.
int if; (incorrect)
int while; (incorrect)
C++ Literals
Literals are data used for representing fixed values. They can be used directly in the code. For example: 1
, 9.5
, 'e'
etc.
Integer Literals
An integer is a numeric literal without any fractional or exponential part.
There are three types of integer literals in C++ programming, An integer literal can be a decimal, octal, or hexadecimal constant. A prefix specifies the base or radix: 0x or 0X for hexadecimal, 0 for octal, and nothing for decimal.
An integer literal can also have a suffix that is a combination of U and L, for unsigned and long, respectively. The suffix can be uppercase or lowercase and can be in any order.
0, -9, 22 etc //Decimal
021, 077, 033 etc //Octal
0x7f, 0x2a, 0x521 etc //Hexadecimal
30 // int
30u // unsigned int
30l // long
30ul // unsigned long
Floating-point Literals
A floating-point literal has an integer part, a decimal point, a fractional part, and an exponent part. You can represent floating point literals either in decimal form or exponential form.
While representing using decimal form, you must include the decimal point, the exponent, or both and while representing using exponential form, you must include the integer part, the fractional part, or both. The signed exponent is introduced by e or E.
3.14159 // Legal
314159E-5L // Legal
510E // Illegal: incomplete exponent
210f // Illegal: no decimal or exponent
.e55 // Illegal: missing integer or fraction
Boolean Literals
There are two Boolean literals and they are part of standard C++ keywords
- A value of true representing true.
- A value of false representing false.
You should not consider the value of true equal to 1 and value of false equal to 0.
Characters
A character literal is created by enclosing a single character inside single quotation marks. For Example: 'a'
, 'm'
, 'F'
, '2'
, '}'
etc.
Escape Sequences
Sometimes, it is necessary to use characters that cannot be typed or has special meaning in C++ programming. For example, newline (enter), tab, question mark, etc.
In order to use these characters, escape sequences are used.
Escape Sequences | Characters |
---|---|
\b | Backspace |
\f | Form feed |
\n | Newline |
\r | Return |
\t | Horizontal tab |
\v | Vertical tab |
\\ | Backslash |
\' | Single quotation mark |
\" | Double quotation mark |
\? | Question mark |
\0 | Null Character |
Following is the example to show a few escape sequence characters −
#include <iostream>
using namespace std;
int main() {
cout << "It's a great\tday\n\nto start your journey";
return 0;
}
When the above code is compiled and executed, it produces the following result −
It's a great day
to start your journey
String Literals
String literals are enclosed in double quotes. A string contains characters that are similar to character literals: plain characters, escape sequences, and universal characters.
“hello ,dear” | string constant |
"" | null string constant |
" " | string constant of six white space |
"y" | string constant having a single character |
"Anybody Can Learn\n" | prints string with a newline |
C++ Constants
The const keyword is a qualifier, it changes the behavior of the variable and makes it a read-only type.
#include <iostream>
using namespace std;
int main() {
const int LENGTH = 10;
const int WIDTH = 5;
const int WIDTH = 5; // Error! WIDTH is a constant.
int area;
area = LENGTH * WIDTH;
cout << area;
cout << NEWLINE;
return 0;
}
Here, we have used the keyword const
to declare a constant named WIDTH
. If we try to change the value of WIDTH
, we will get an error.
A constant can also be created using the #define
preprocessor directive. Let’s understand with an example.
#include <iostream>
using namespace std;
#define LENGTH 10
#define WIDTH 5
int main() {
int area;
area = LENGTH * WIDTH;
cout << area;
return 0;
}