Table of Contents
In this article, you’ll learn How to read user or console input in PHP.
What is a PHP console?
A PHP console is a command-line interface for writing and executing PHP codes. A PHP console is usually called the PHP interactive shell.
If you have PHP installed, you can access the console with the terminal command below:
php -a
However, it is more difficult to get user input through the PHP console as compared to using web browsers for input.
Prompting users for input
To get input from users, you also have to prompt them to enter something. You can use PHP’s `readline() function to get this input from the console.
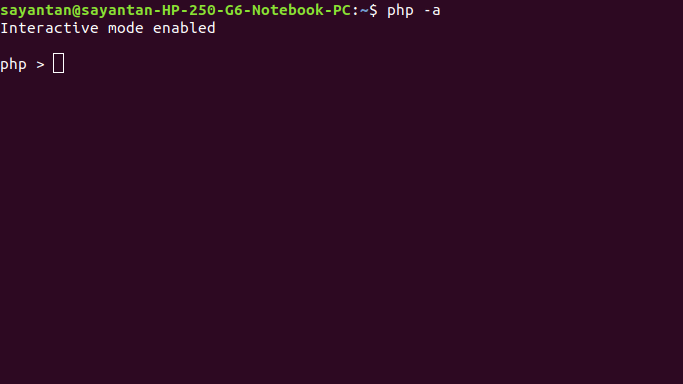
What is the readline()
function?
readline()
is a built-in function in PHP that enables us to read input from the console. The function accepts an optional parameter that contains text to display.
Syntax
readline('input your name');
Code
The code below shows how you can read a single input from the user.
<?php
$name = (string)readline("Your name: ");
$intergernumber= (int)readline('Enter an integer: ');
$floatnumber = (float)readline('Enter a floating point number: ');
echo "Hello $name The integer value you entered is $intergernumber and the float value is $floatnumber";
?>
Output
Your name: Anup Maurya Enter an integer: 23 Enter a floating point number: 34.34 Hello Anup Maurya The integer value you entered is 23 and the float value is 34.34
Accepting multiple inputs
The readline()
function can also help you accept multiple inputs, which are separated by some delimiter.
To achieve this, you must use another function called explode()
together with readline()
. The first argument of explode()
is the delimiter you want to use. The second argument will be the readline()
function.
The code below shows how to accept multiple inputs from the console:
<?php
// For input
// 0 1 2 3 4 5 6
echo "Enter a multiple space separated values: ";
$arr = explode(' ', readline());
// For output
print_r($arr)
?>
Output
Enter a multiple space separated values: 23 34 23 24 64 21 56 6 Array ( [0] => 23 [1] => 34 [2] => 23 [3] => 24 [4] => 64 [5] => 21 [6] => 56 [7] => 6 )
That’s all in this article of How to read user or console input in PHP.