Table of Contents
In this article, you can look at Accenture Coding Questions for Placements that are asked in various years.
- In Accenture there will be 2 or 3 coding questions that you have to solve in 45 minutes.
- In the Accenture Coding Round ,you can write coding using in these preferred language.
a. C
b. C++
c. Java
d. Python
The difficulty level of the questions are medium. You have to practice a lot to get a good score in the Accenture coding Questions.
Rules for Accenture Coding Questions Section
- Two questions will be asked. You’ve to solve both the questions in 45 minutes.
- You must start your code from scratch.
- The Coding section is divided into two, first for writing the code and the other for the output. So you should write the whole program.
- Errors are clearly displayed.
Accenture Coding Questions With Solutions 2021, 2022 & 2023
Problem Statement 1
Write a function SmallLargeSum(array) which accepts the array as an argument or parameter, that performs the addition of the second largest element from the even location with the second largest element from an odd location?
Rules:
a. All the array elements are unique.
b. If the length of the array is 3 or less than 3, then return 0.
c. If Array is empty then return zero.
Sample Test Case 1:
Input:
6
3 2 1 7 5 4
Output:
7
Explanation: The second largest element in the even locations (3, 1, 5) is 3. The second largest element in the odd locations (2, 7, 4) is 4. So the addition of 3 and 4 is 7. So the answer is 7.
Sample Test Case 2:
Input:
7
4 0 7 9 6 4 2
Output:
10
Solution:
- First we’ve to take input from the user i.e in the main function.
- Then we’ve to create two different arrays in which the first array will contain all the even position elements and the second one will contain odd position elements.
- The next step is to sort both the arrays so that we’ll get the second-largest elements from both the arrays.
- At last, we’ve to add both the second-largest elements that we get from both the arrays.
- Display the desired output in the main function.
Code Implementation:
#include <bits/stdc++.h>
using namespace std;
int smallLargeSum(int *arr, int n) {
if(n <= 3) {
return 0;
}
//Here we use vector because we don't know the array size,
//we can use array also but vector gives us more functionality than array
vector<int> arrEven, arrOdd;
//Break array into two different arrays even and odd
for(int i = 0; i < n; i++) {
//If Number is even then add it into even array
if(i % 2 == 0) {
arrEven.push_back(arr[i]);
}
else {
arrOdd.push_back(arr[i]);
}
}
//Sort the even array
sort(arrEven.begin(), arrEven.end());
//We use sort function from C++ STL library
//Sort the odd array
sort(arrOdd.begin(), arrOdd.end());
//Taking second largest element from both arrays and add them
return arrEven[1] + arrOdd[1];
}
// Driver code
int main()
{
int n;
cout<<"Enter How many elements you want to enter?\n";
cin>>n;
int arr[n];
//Get input from user
cout<<"Start entering the numbers\n";
for(int i = 0; i < n; i++) {
cin>>arr[i];
}
cout<<"Output is\n";
cout<<smallLargeSum(arr, n);
return 0;
}
Problem Statement 2
Write a function CheckPassword(str) which will accept the string as an argument or parameter and validates the password. It will return 1 if the conditions are satisfied else it’ll return 0?
The password is valid if it satisfies the below conditions:
a. It should contain at least 4 characters.
b. At least 1 numeric digit should be present.
c. At least 1 Capital letter should be there.
d. Passwords should not contain space or slash(/).
e. The starting character should not be a number.
Sample Test Case:
Input:
bB1_89
Output:
1
Approach:
- Using if condition check whether the length of string is greater than equal to 4 or not.
- Run a loop on the string and check if any character is a digit or not. It is a digit if it’s between ‘0’ and ‘9’.
- Iterate the string and check that only at least one letter should be between ‘A’ and ‘Z’, i.e it should be capital.
- Run a loop on string and check no character should match space(‘ ‘) or slash (‘/’).
- Check that the first character should not lie between ‘0’ and ‘9’.
Code Implementation
#include<iostream>
#include<string.h>
using namespace std;
int CheckPassword(char str[]) {
int len = strlen(str);
bool isDigit = false, isCap = false, isSlashSpace=false,isNumStart=false;
//RULE 1: At least 4 characters in it
if (len < 4)
return 0;
for(int i=0; i<len; i++) {
//RULE 2: At least one numeric digit in it
if(str[i]>='0' && str[i]<='9') {
isDigit = true;
}
//RULE 3: At least one Capital letter
else if(str[i]>='A'&&str[i]<='Z'){
isCap=true;
}
//RULE 4: Must not have space or slash
if(str[i]==' '|| str[i]=='/')
isSlashSpace=true;
}
//RULE 5: Starting character must not be a number
isNumStart = (str[0]>='0' && str[0]<='9');
//FYI: In C++, if int data type function returns the true then it prints 1 and if false then it prints 0
return isDigit && isCap && !isSlashSpace && !isNumStart;
}
int main() {
char password[100];
cout<<"Enter the Password\n";
cin>>password;
cout<<"The output is =\n";
cout<<CheckPassword(password);
}
Problem Statement 3
A person’s body mass index is a simple calculation based on height and weight that classifies the person as underweight, overweight, or normal. The formula for metric unit is,
BMI = weight in kilograms / (height in meters)2
You are given a function,
Int GetBMICategory(int weight, float height);
The function accepts the weight (an integer number) and height (a floating point number) of a person as its arguments. Implement the function such that it calculations the BMI of the person and returns an integer, the person’s BMI category as per the given rules:
- If BMI < 18, return 0.
- If 18 >= BMI < 25, return 1.
- If 25 >= BMI <30, return 2.
- If 30 >= BMI < 40, return 3.
- If BMI >= 40, return 4.
Note:
- Weight > 0 and its unit is kilogram.
- Height > 0 and its unit is metre.
- Compute BMI as a floating-point.
Sample Test Case:
Input:
42
1.54
Output:
0
Explanation:
The person’s weight is 42Kg and height is 1.54 metres BMI = weight in kilograms / (height in meters)2 = 42/(1.54 * 1.54) = 17.70
Since, BMI < 18, as per given rules, the output is 0.
Sample Input:
62
1.52
Sample Output:
2
Code Implementation
// BMI Problem statement
#include<iostream>
using namespace std;
int bmi(int weight, float height){
float ans=weight/(height*height);
if(ans<18)
return 0;
else if(ans>=18 && ans<25)
return 1;
else if(ans>=25 && ans<30)
return 2;
else if(ans>=30 && ans<40)
return 3;
else
return 4;
}
int main(){
int weight;
float height;
cin>>weight>>height;
cout<<bmi(weight,height);
}
Problem Statement 4 : Asked on [05/2021]
DICE SUM: You are given 2 unbiased dice containing 6 faces each. And also you are given with a “sum” which should be obtained by throwing those two dice at same time. You have to print the number of possibilities where the sum of both dice is equal to output sum. If there are no possibilities return zero.
For Example:
Input
10
Output
3
Explaination
Possible outcomes with sum 10 are (5,5), (6,4) and (4,6).
Problem Statement 5 : Asked on [07/2023]
A start up wants to expand their empire so the CEO decided to purchase plots at various locations. He has already figured out some plots and now he is busy, so he wants that you will select some plots that are square shaped.
Write a code, to help your CEO for finding the all square shaped Plots.
Input:
First line of input will have the total shortlisted plots by the CEO
Second line represents N space separated integers denoting the area of the plots.
Output:
Find out how many plots are there to build a new office
Example:
Input: 6
64 16 38 81 50 100
Output: 3
Problem Statement 6 : Asked on [07/2023]
Find Maximum Element and its index, A function accepts an array and length, implement the function to find the maxil of array and print mximum element and its index.
The maximum element and its index should be printed in next line.
Conditions:
- Array index starts with O
- Maximum element and its index should be separated by new line.
- Assume there is only 1 maximum element in array.
Sample Input:
1 2 3 4 5 6 7
Output:
7
6
Example 2:
23 45 82 71
output :
82
2
Problem Statement 7 : Asked on [07/2023]
You have given an integer number N determine the number of bricks needed to make a house of brick of N levels.
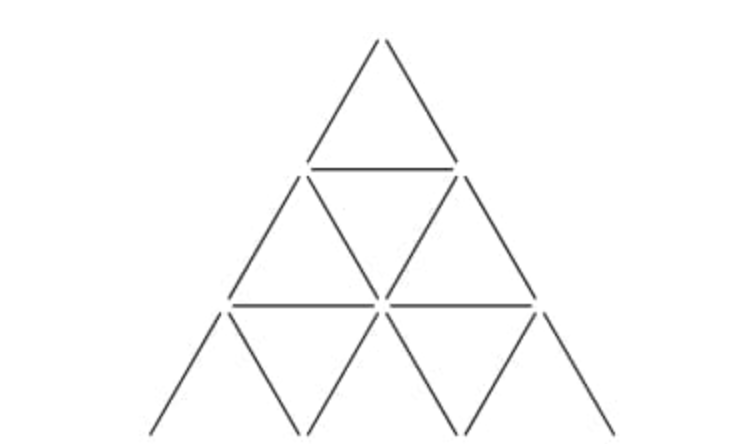
Input:
1
Output:
2
Input:
3
Output:
15
Code Implementation
#include <iostream>
using namespace std;
int
main ()
{
int n;
cin >> n;
int ans = n * (3 * n + 1) / 2;
cout << ans;
return 0;
}
Problem Statement 8 : Asked on [07/2023]
You are given a set of numbers and you can arrange them in any order but this pays a penalty equal to the sum of the absolute differences between contiguous numbers
You are needed to return the minimum penalty that should be paid.
Input Format
Input 1: Size of the array of integer numbers (2<input< 10001)
Input 2: Integer Array (1<=input<=10000 )
Example 1:
Input 1: 3
Input 2: {1,3,2}
Output: 2
Problem Statement 9 : Asked on [07/2023]
Write an algorithm to determine if a number n is round number.
A round number is a number defined by the following process:
Starting with any positive integer, replace the number by the sum of the squares of its digits.
Repeat the process until the number equals 1 (where it will stay), or it loops endlessly in a cycle which does not include 1.
Those numbers for which this process ends in 1 are happy.
Return true if n is a happy number, and false if not.
Input:
n = 19
Output:
true
Explanation:
1^2+ 9^2 = 82
8^2 + 2^2= 68
6^2 + 8^2 = 100
1^2 + 0^2 + 0^2 = 1
Problem Statement 10 : Asked on [10/2023]
Alex is given a number N. He has to check whether the number is a Meta number or not. Meta numbers are the numbers that are divisible by 1, 2, 4 and B but not by 10. Your task is to find the number of Meta Numbers in the range 1 to N.
Input format:
The input consists of a single line:
- The line contains a single integer N
- The input will be read from the STDIN by the candidate
Output format:
Print the number of Meta Numbers in the range 1 to N.
The output will be matched to the candidate’s output printed on the STDOUT
Constraints:
1 N≤ 109
Example:
Input:
20
Output:
2
Explanation:
8 and 16 are meta numbers as they are divisible by 1, 2, 4 and 8 but are not divisible by 10.
Code Implementation
#include <bits/stdc++.h>
using namespace std;
int Count_Meta (int N){
int count=0;
for (int 1=1;1<=N;i++){
if(1%1==0 && 1%2==0 && 1%4==0 && 1%8==0 && 1%10!=0){
count++;
}
}
return count;
}
int main() {
int n;
cin>>n;
int ans=Count_Meta(n);
cout<<ans;
return 0;
}