Table of Contents
A React element is like a set of instructions for creating a part of a web page. Instead of directly giving instruction to the web page itself, React uses a kind of blueprint called the “virtual DOM.” This blueprint is made up of these React elements, which are like special building blocks. They look a bit like the parts of a webpage, but they’re actually written in a special type of JavaScript.
Think of React elements as if you’re telling a browser, “Hey, make this part of the webpage look like this!” You make these instructions using a mix of regular HTML stuff and some special JavaScript code.
So, when you want to show something on a webpage using React, you use these React elements to give the browser the right instructions on what to display.
React Element
We create the react elements using the below syntax by embedding HTML elements in JavaScript to display the content on the screen.
React.createElement(type,{props},children);
React.createElement() takes three arguments. They are as follows
- type: the type of the HTML element (h1,p,button).
- props: properties of the object({style:{size:10px}} or Eventhandlers, classNames,etc).
- children: anything that need to be displayed on the screen.
ReactDOM
ReactDOM contains the arguments that are necessary to render react elements in the browser.
ReactDOM.render(element,containerElement);
ReactDOM.render() takes two arguments. They are as follows
- element: The element that needs to be rendered in the DOM.
- containerElement: Where to render in the dom.
A React Application
A React Application using React CDN to include react and reactDOM inside the project.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>A React Application - Anup Maurya</title>
</head>
<body>
<div id="root"></div>
</body>
<script crossorigin src="https://unpkg.com/react@18/umd/react.development.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@18/umd/react-dom.development.js"></script>
<script>
const heading =React.createElement("h1",{},"Namaste Everyone!");
const root =ReactDOM.createRoot(document.getElementById("root"));
root.render(heading);
</script>
</html>
Output:
Output is visible in your browser.
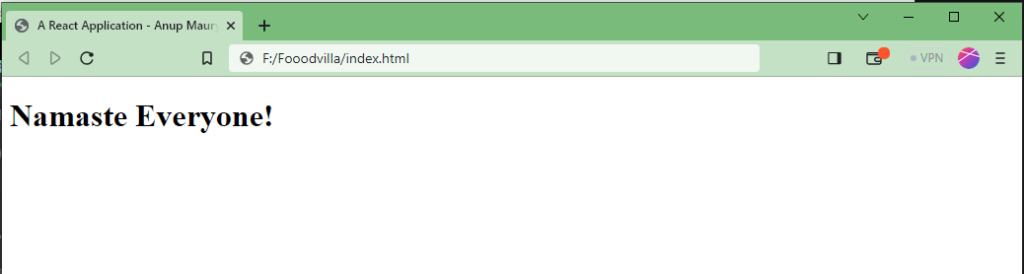