Table of Contents
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
Encapsulation
Encapsulation can be defined as wrapping up of data members and member functions in a single entity, encapsulation is done for data hiding or information hiding, by using encapsulation we can make our class “Read Only”. fully encapsulated class is a class where all data members are private, given below is a simple demonstration of Encapsulation.
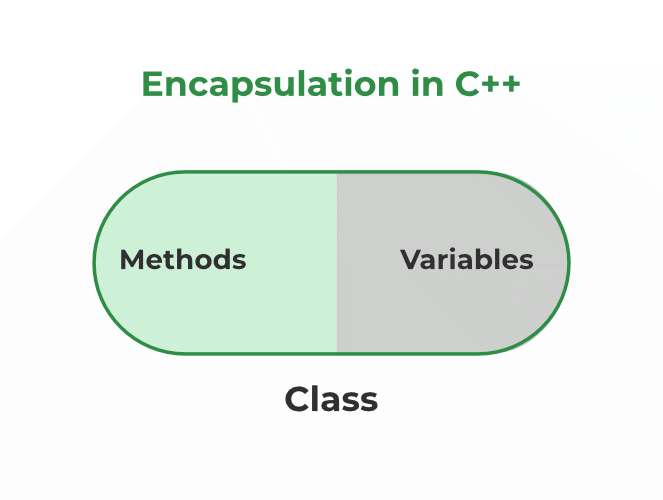
#include<iostream>
using namespace std;
class Secret{
private:
int rank=5;
public:
//getter function
int getRank(){
return rank;
}
};
int main(){
Secret person1;
cout<<person1.getRank()<<endl;
return 0;
}
The above code follow the “read only” feature of Encapsulation, since we can not modify data members we can only read it by getter( ) function.
Inheritance
The capability of a class to derive or inherit properties or characteristics from another class defines the Inheritance, Inheritance is one of the most important features of OOPs.
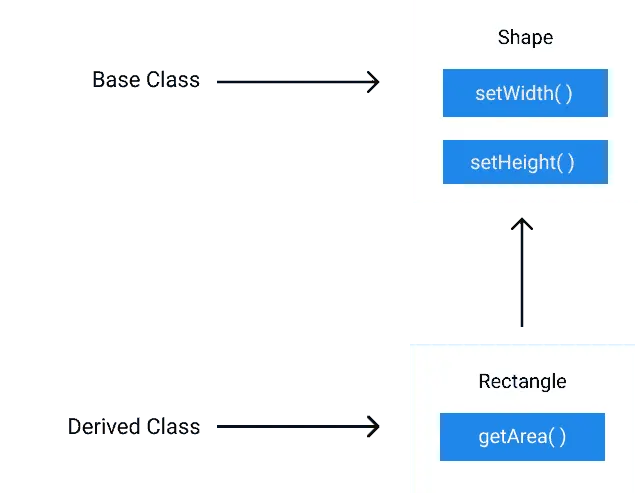
Given below is a simple demonstration of Inheritance
#include<iostream>
using namespace std;
class Parent{
private:
int rank=5;
char ID='A';
public:
int getRank(){
return rank;
}
};
class Child:public Parent{
public:
string name;
void setName(string name){
this->name=name;
}
};
int main(){
Child obj;
cout<<obj.getRank()<<endl;
return 0;
}
Output
5
Here Child is a derived class and Parent is a base class.
Mode of Inheritance
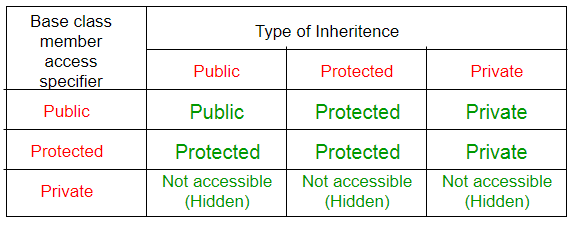
Above table shows how the child class can use the data members of the base class, a child class can never access the private data members of base class even if the type of Inheritance is Public, Protected or Private, hence we can conclude that a Private data member of any class can’t be Inherited.
Type of Inheritance
- Single Inheritance
- Multi-level Inheritance
- Multiple Inheritance
- Hybrid Inheritance
- Hierarchical inheritance
1) Single Inheritance
Single Inheritance is all about one subclass and one base class, basically in Single Inheritance the class is allowed to Inherit properties from only one class.
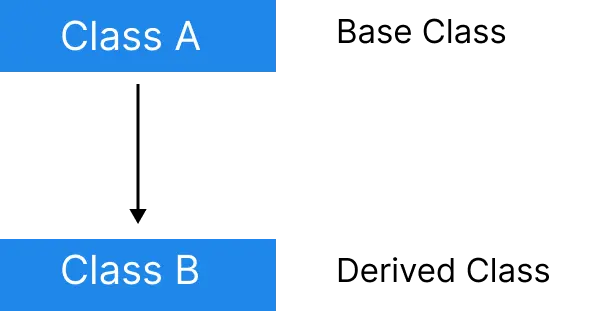
// inheritance.cpp
#include <iostream>
using namespace std;
class base //single base class
{
public:
int x;
void getdata()
{
cout << "Enter the value of x = "; cin >> x;
}
};
class derive : public base //single derived class
{
private:
int y;
public:
void readdata()
{
cout << "Enter the value of y = "; cin >> y;
}
void product()
{
cout << "Product = " << x * y;
}
};
int main()
{
derive a; //object of derived class
a.getdata();
a.readdata();
a.product();
return 0;
}
Output
Enter the value of x = 25 Enter the value of y = 15 Product = 375
2) Multi-level Inheritance
In multi-level Inheritance a derived class is defined from another derived class.
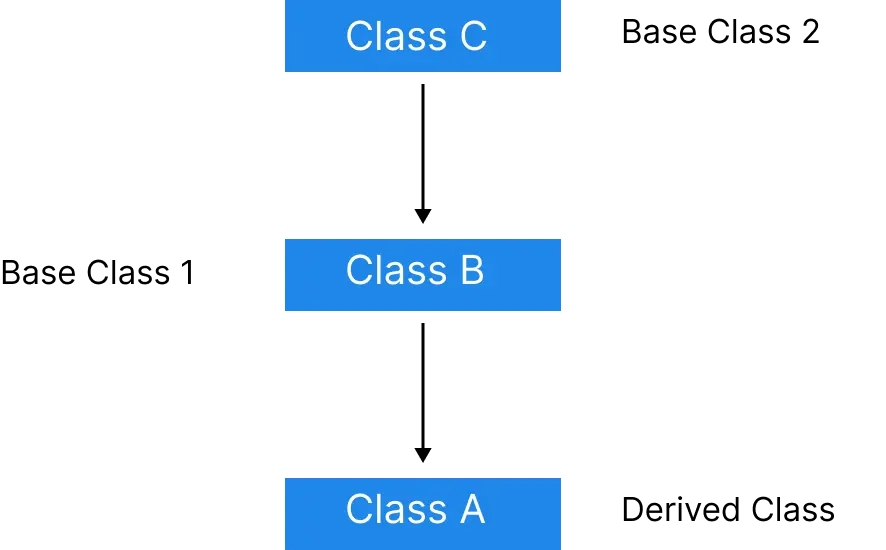
// inheritance.cpp
#include <iostream>
using namespace std;
class base //single base class
{
public:
int x;
void getdata()
{
cout << "Enter value of x= "; cin >> x;
}
};
class derive1 : public base // derived class from base class
{
public:
int y;
void readdata()
{
cout << "\nEnter value of y= "; cin >> y;
}
};
class derive2 : public derive1 // derived from class derive1
{
private:
int z;
public:
void indata()
{
cout << "\nEnter value of z= "; cin >> z;
}
void product()
{
cout << "\nProduct= " << x * y * z;
}
};
int main()
{
derive2 a; //object of derived class
a.getdata();
a.readdata();
a.indata();
a.product();
return 0;
}
Output
Enter value of x= 58 Enter value of y= 64 Enter value of z= 48 Product= 178176
3) Multiple Inheritance
Multiple Inheritance is concept in which a class can inherit properties and features from more than one class, in multiple Inheritance more than one base class are present.
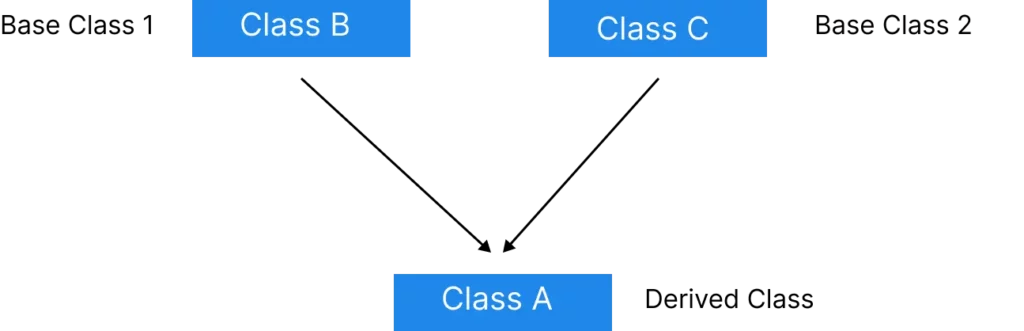
// multiple inheritance.cpp
#include<iostream>
using namespace std;
class A
{
public:
int x;
void getx()
{
cout << "enter value of x: "; cin >> x;
}
};
class B
{
public:
int y;
void gety()
{
cout << "enter value of y: "; cin >> y;
}
};
class C : public A, public B //C is derived from class A and class B
{
public:
void sum()
{
cout << "Sum = " << x + y;
}
};
int main()
{
C obj1; //object of derived class C
obj1.getx();
obj1.gety();
obj1.sum();
return 0;
}
Output
enter value of x: 54 enter value of y: 965 Sum = 1019
4) Hierarchical inheritance
In hierarchical Inheritance more than one sub class is Inherited from single parent class or base class.
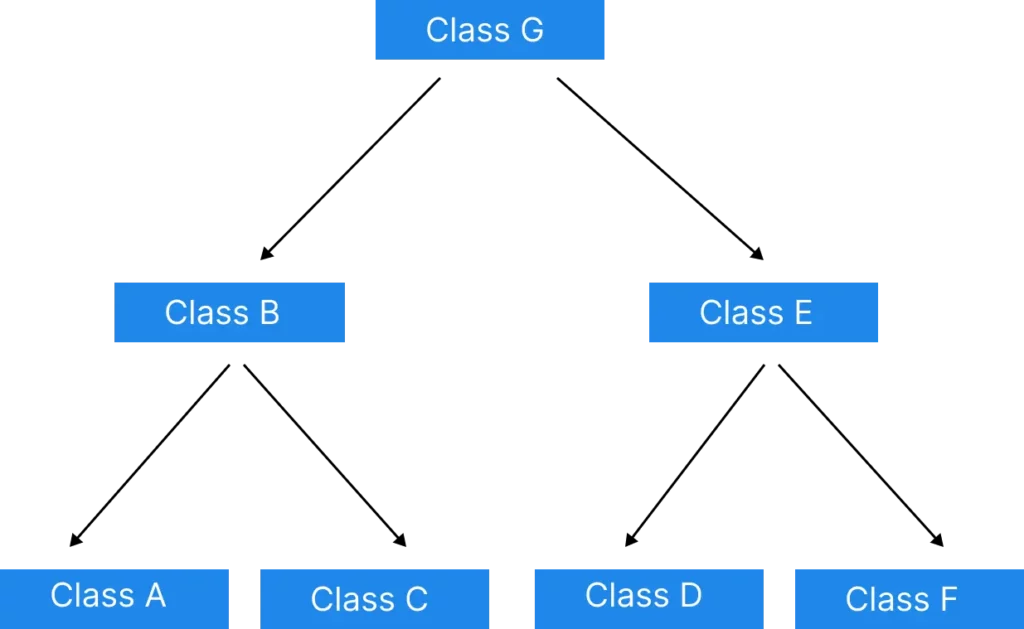
// hierarchial inheritance.cpp
#include <iostream>
using namespace std;
class A //single base class
{
public:
int x, y;
void getdata()
{
cout << "\nEnter value of x and y:\n"; cin >> x >> y;
}
};
class B : public A //B is derived from class base
{
public:
void product()
{
cout << "\nProduct= " << x * y;
}
};
class C : public A //C is also derived from class base
{
public:
void sum()
{
cout << "\nSum= " << x + y;
}
};
int main()
{
B obj1; //object of derived class B
C obj2; //object of derived class C
obj1.getdata();
obj1.product();
obj2.getdata();
obj2.sum();
return 0;
}
Output
Enter value of x and y: 45 45 Product= 2025 Enter value of x and y: 48 65 Sum= 113
5) Hybrid Inheritance:
Hybrid Inheritance is basically an implementation which is using more than one type of Inheritance, In the above diagram the multilevel inheritance and hierarchical Inheritance is used.
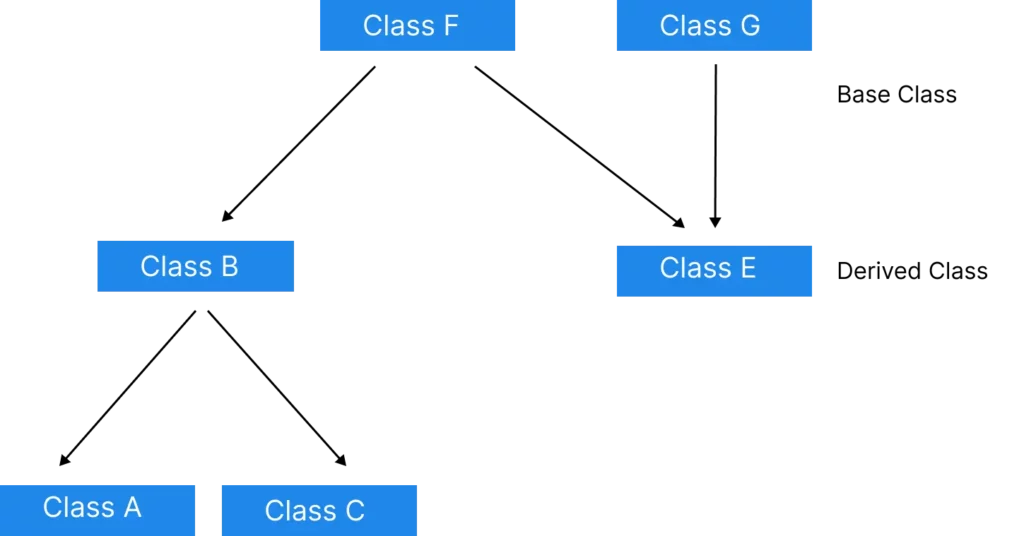
#include <iostream>
using namespace std;
class A
{
public:
int x;
};
class B : public A
{
public:
B() //constructor to initialize x in base class A
{
x = 10;
}
};
class C
{
public:
int y;
C() //constructor to initialize y
{
y = 4;
}
};
class D : public B, public C //D is derived from class B and class C
{
public:
void sum()
{
cout << "Sum= " << x + y;
}
};
int main()
{
D obj1; //object of derived class D
obj1.sum();
return 0;
}
Output
Sum= 14
Polymorphism
The word polymorphism means having many forms. In simple words, we can define polymorphism as the ability of a message to be displayed in more than one form.
An operation may exhibit different behaviours in different instances.
C++ supports operator overloading and function overloading.
- Operator Overloading
#include<iostream>
using namespace std;
class Complex {
private:
int real, imag;
public:
Complex(int r = 0, int i = 0) {real = r; imag = i;}
// This is automatically called when '+' is used with
// between two Complex objects
Complex operator + (Complex const &obj) {
Complex res;
res.real = real + obj.real;
res.imag = imag + obj.imag;
return res;
}
void print() { cout << real << " + i" << imag << '\n'; }
};
int main()
{
Complex c1(10, 5), c2(2, 4);
Complex c3 = c1 + c2;
c3.print();
}
- Function Overloading
#include <iostream>
using namespace std;
void add(int a, int b)
{
cout << "sum = " << (a + b);
}
void add(double a, double b)
{
cout << endl << "sum = " << (a + b);
}
int main()
{
add(10, 2);
add(5.3, 6.2);
return 0;
}
Abstraction
Abstraction is used for implementation hiding, it is one of the most important features of OOPs, Abstraction is basically showing only essential information and hiding the details of the program.
For example: while using a online banking mobile application the user can easily make a payment without any access of the backend of the application, this way Abstraction can be utilised for security purposes.
// C++ Program to Demonstrate the
// working of Abstraction
#include <iostream>
using namespace std;
class implementAbstraction {
private:
int a, b;
public:
// method to set values of
// private members
void set(int x, int y)
{
a = x;
b = y;
}
void display()
{
cout << "a = " << a << endl;
cout << "b = " << b << endl;
}
};
int main()
{
implementAbstraction obj;
obj.set(10, 20);
obj.display();
return 0;
}
Output
a = 10 b = 20